Converter는 데이터 형식을 변환할 때 사용됩니다. WPF(Windows Presentation Foundation)를 사용하다보면 XAML에서 데이터를 변환해야 하는 경우가 많이 있습니다. 이번 포스팅에서는 Converter가 무엇이며 사용법에 대해 정리하고자 합니다.
Converter란 무엇일까?
Converter는 데이터 바인딩(Data Binding) 중에 데이터 값을 다른 형식으로 변환시키는 역할을 합니다. 바인딩된 속성 값을 변환하여 UI(User Interface)에 보여주거나, UI에서 입력 받은 값을 변환하는데 사용됩니다. 예를 들어 사용자로부터 입력받은 문자열을 숫자로 변환하여 전달할 수 있습니다.
Converter 사용 방법
Converter를 사용하기 위해서는 Converter Class 생성, XAML에서 연결, 매개변수 설정 3가지 단계로 정리할 수 있습니다.
Converter Class 생성
Converter를 사용하기 위해서는 IValueConver 인터페이스를 구현한 Class를 생성합니다. 해당 인터페이스에는 Convert, ConvertBack 라는 2개의 메서드를 포함하고 있으며 Convert는 데이터 값을 UI에 표현할 형식 변환, ConvertBack은 UI에서 입력받은 값을 데이터 값에 맞게 변환하는 역할을 합니다.
class MyConverter : IValueConverter
{
public object Convert(object value, Type targetType, object parameter, CultureInfo culture)
{
if (value.ToString() != string.Empty)
return true;
else
return false;
}
public object ConvertBack(object value, Type targetType, object parameter, CultureInfo culture)
{
throw new NotImplementedException();
}
}
XAML에서 연결
생성한 Converter Class를 사용하기 위해서는 XAML에서 정의하고 데이터 바인딩(Data Binding) 시에 Converter를 연결해야 합니다. 이를 위해서는 Binding 요소에서 Converter 속성을 사용해야 합니다.
<Window x:Class="WPFExample.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:local="clr-namespace:WPFExample"
mc:Ignorable="d"
Title="MainWindow" Height="100" Width="220">
<Window.Resources>
<local:MyConverter x:Key="myConverter"/>
</Window.Resources>
<StackPanel VerticalAlignment="Center">
<TextBox x:Name="tbk" Width="200" Height="20" Text=""/>
<Button x:Name="btn" Content="Button" Width="200" IsEnabled="{Binding ElementName=tbk, Path=Text,
Mode=OneWay, Converter={StaticResource myConverter}}"/>
</StackPanel>
</Window>
매개변수 설정
Converter에는 매개변수를 설정할 수 있으며 이를 통해서 UI에서 데이터 변환 시에 추가 정보를 전달할 수 있습니다. 이를 위해서는 ConverterParameter 속성을 사용해야 합니다.
Converter 예제
<Window x:Class="WPFExample.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:local="clr-namespace:WPFExample"
mc:Ignorable="d"
Title="MainWindow" Height="100" Width="220">
<Window.Resources>
<local:MyConverter x:Key="myConverter"/>
</Window.Resources>
<StackPanel Orientation="Horizontal" HorizontalAlignment="Center">
<ComboBox x:Name="cbx" ItemsSource="{Binding}" Width="60" Height="20">
</ComboBox>
<Rectangle Width="50" Height="50" Fill="{Binding ElementName=cbx, Path=SelectedIndex, Mode=OneWay, Converter={StaticResource myConverter}}" Margin="5" />
</StackPanel>
</Window>
namespace WPFExample
{
public static class MyData
{
public enum MyColor
{
Red,
Green,
Blue,
}
}
}
using System;
using System.Globalization;
using System.Windows.Data;
using System.Windows.Media;
namespace WPFExample
{
class MyConverter : IValueConverter
{
public object Convert(object value, Type targetType, object parameter, CultureInfo culture)
{
if (value != null)
{
switch ((MyData.MyColor)value)
{
case MyData.MyColor.Red:
return new SolidColorBrush(Colors.Red);
case MyData.MyColor.Green:
return new SolidColorBrush(Colors.Green);
case MyData.MyColor.Blue:
return new SolidColorBrush(Colors.Blue);
default:
break;
}
}
return Colors.White;
}
public object ConvertBack(object value, Type targetType, object parameter, CultureInfo culture)
{
throw new NotImplementedException();
}
}
}
using System;
using System.Collections.Generic;
using System.Linq;
using System.Windows;
using System.Windows.Controls;
namespace WPFExample
{
public partial class MainWindow : Window
{
public MainWindow()
{
InitializeComponent();
List<string > colors = new List<string>();
colors = Enum.GetNames(typeof(MyData.MyColor)).ToList();
this.DataContext = colors;
}
}
}
ComboBox에서 Item을 선택하게 되면 선택한 item index 정보는 Converter에 전달됩니다. ComboBox의 item들은 Enum Colors를 list 형식으로 변환하여 반영했기 때문에 Converter는 전달받은 index 값을 Enum Colors와 비교하여 일치하는 색상으로 변환합니다. 변환된 색상 값은 Rectangle의 Fill에 반영됩니다.
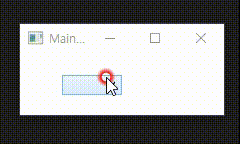